SHA-256 in a JavaScript application to integrate with an API
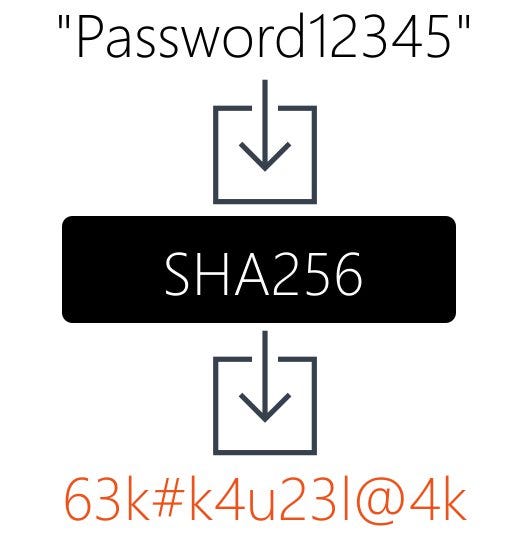
Here is an example of using SHA-256 in a JavaScript application to integrate with an API:
// Import the crypto library
const crypto = require('crypto');
// Function to calculate the SHA-256 hash of a string
function calculateHash(data) {
return crypto.createHash('sha256').update(data).digest('hex');
}
// Example API request function
function sendAPIRequest(data) {
// Calculate the SHA-256 hash of the request data
const hash = calculateHash(data);
// Add the hash to the request headers
const headers = { 'Content-Type': 'application/json', 'X-Data-Hash': hash };
// Send the API request with the request data and headers
fetch('https://api.example.com/transactions', {
method: 'POST',
headers: headers,
body: JSON.stringify(data)
})
.then(response => {
// Get the X-Data-Hash header from the response
const receivedHash = response.headers.get('X-Data-Hash');
// Compare the received hash to the calculated hash of the response data
if (receivedHash === calculateHash(response.data)) {
console.log('Response data integrity verified.');
// Use the response data as needed
} else {
console.error('Response data integrity check failed.');
}
})
.catch(error => {
console.error('API request failed:', error);
});
}
// Example usage
const transactionData = { account: '123456', amount: 100.50 };
sendAPIRequest(transactionData);
This example uses the crypto library in Node.js to calculate the SHA-256 hash of the request data and response data. It also shows how to include the hash in the request headers and how to check the integrity of the response data by comparing the received hash with the calculated hash of the response data.